Blog » Coding
Researching Open Source Javascript + Front End Tools, 2013
Intent
In 2013, I took a short career break to recharge. During this time I spent valuable time with my late father, began interning as a brewer's apprentice at Metropolitan Brewing, built a standing desk in my home office, and invested several months researching emerging open source javascript and front-end technologies. I used my portfolio project as an outlet and guinea pig for this experimentation. This blog post is a summary of the insights I gained from that research.
Touch points include working environments, build tools, client side application development, testing, deployment and a few frameworks. Several of which were either open source, or created for the open source community. Technologies integrated include: GruntJS, AngularJS, Karma Runner, AssembleJS, Yeoman, Bower, CoffeeScript, Bootstrap, Foundation, SASS, Jasmine, Protractor, Jenkins, Travis and more.
The portfolio project source is on GitHub, and the demo version is up on GitHub Pages. I inject my own data into this framework, and serve the personalized version mostly privately. The code and practices described below will ultimately become outdated, obsolete, or refactored out. Alas, this is the reality of technology.
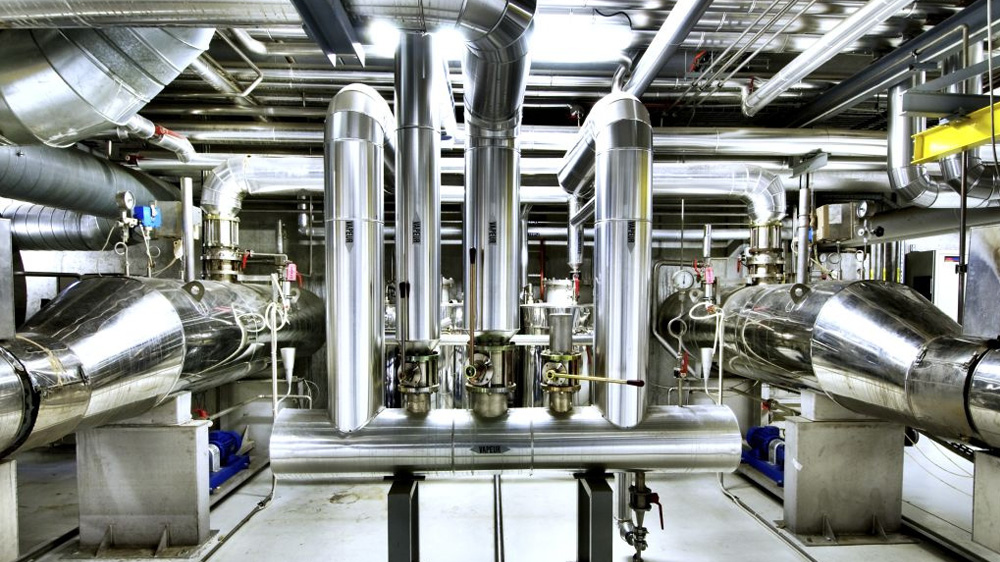
Workflow
- Command Line Interface. Over the past few years, I began noticing a trend in web community favoring the command line interface (CLI). Wanting to find out why, I started hacking my shell.
- Installed the Homebrew package manager, and oh-my-zsh to customize my shell.
- Installed rvm to manage Ruby versions, nvm to manage NodeJS versions - and got familiar with installing project related Ruby gems/gemfiles and npm dependencies.
- I now know just enough on the command line to be a danger to myself.
- Yeoman. Explored Google's Yeoman tool set and workflow to scaffold out a base template via its generators. Yeoman introduced me to Grunt and Bower. Soon after I started following Paul Irish, Addy Osamni and Sindre Sorhus.
- Grunt. Javascript task runner CLI tool used to build, preview and test projects. With a bit of learning curve, my Gruntfile has become the cornerstone of this project.
- Bower. Came to appreciate using Twitter's Bower CLI tool for front-end script dependency management. It is now my preferred way to manage front-end libraries. If a library isn't in bower, I politely request the author to register it.
- Editing. Leveraged both Sublime and IntelliJ for editing. Sublime is great for lightweight editing, but I became frustrated with hit-or-miss plugin support. IntelliJ has many more coding comfort features and has become my primary front-end editor.
Scripting
- AngularJS. Angular is Google's answer to front end Javascript application development, and is considered an MVW approach for declaratively and dynamically extending HTML capabilities.
- Using Javascript and AngularJS could have been entirely avoided on this site for a simpler all HTML approach. However, one of my objectives was to become more familiar with Angular for building web applications, so this was a good guinea pig project for that.
- Leveraged Angular primarily in the "work" section for a single page load, dynamic in-browser, low-latency website.
- ShowdownJS has been coupled with the dynamic work section content for ease of Markdown editing.
- CoffeeScript. Applied CoffeeScript (CS) to compact and simplify Javascript code.
- CoffeeScript while concise, does occasionally get difficult to debug if whitespace tabbing gets misaligned.
- Within the Node community, there seems to be some a preference to avoid using CS. Perhaps because of it's need for an additional build step which encumbers quick open source project tweaking.
- Enabling source maps helps with debugging, but getting the maps to be recognized by the browsers can sometimes be hit or miss (this should stabilize over time).
- I remain flexible to using native Javascript, coupled with JSHint.
Photo: HarshLight, Flickr
Scaffolding
- Static Site Generation. The idea behind static site generators is that if you can streamline your website into being just a collection of static HTML files, you can eliminate the need for middleware technologies such as PHP, .NET or Wordpress. In turn, your site will load faster, is easier to maintain and generally cheaper to host. For some application purposes this may not be suitable, but for a generally flat architecture, it works well.
- Initially installed Jekyll and explored Middleman.
- Ended up migrating over to the relatively newer AssembleJS since it met my relatively simple needs, was NodeJS based, and fit snuggling into the Grunt workflow. Assemble also leverages HandlebarsJS. I've been making minor contributions to the project repository - mainly documentation and fixes.
- Front-end Framework.
- Initially applied mobile-first, EM and SASS oriented Zurb Foundation 4 framework.
- Once mobile-first Bootstrap 3 was released, I migrated the project over to Bootstrap.
- Choose Bootstrap mainly because it has such a large following and I wanted to become more familiar with it in case one day I wanted to leverage offshoots like WrapBootstrap.
- Leveraged Bootstrap-SASS port in favor or LESS for use of source maps (as of date of this writing stable release for LESS source maps is not available), and leveraged mix-ins to minimize poluttion of DOM with Bootstrap's styling classes.
- Bootstrap is still PX oriented instead of EM, but I can live with that (for now) given it's not much of a concern for my current target browsers/audience. However, EMs is still a hot topic that hopefully will be addressed in a future Bootstrap release.
- Am also mildly entertained keeping up with the personalities behind Bootstrap: @mdo and @fat.
Image: Nyan Cat / Rob Bulmahn, Flickr
Styling
- Cascade. Applied SASS for CSS meta-scripting and source mapping. Leveraged Node's lib-sass/grunt-sass for faster compliations instead of Ruby based grunt-contrib-sass. Briefly used LESS, Bourbon, Compass and Susy before pulling them out.
- Videos. ScreenFlow allowed me to easily edit together professional HD/1080p quality "walk-through" screen capture videos of my work. I experimented using VideoJS and Vimeo's API to stream the videos in. But ultimately chose to embed with videos through YouTube's JS API because of a greater feature set and ability to load in high resolution default/poster images.
- Imagery. Discovered PhotoDune and GraphicRiver as an amazing and cost effective resource for stock photography and device mock-ups. "Free" creative commons images are also great.
- Fonts. Opted to use Google Fonts over the TypeKit. Also leveraging FontAwesome for icons.
Photo: Jeff Krause, Flickr
Testing
- Development. While authoring, I usually test against Chrome Canary for access to latest dev tools, with grunt-contrib-livereload.
- Behavior Driven Development. For BDD testing, I wrote Jasmine tests for unit and integration testing.
- Units. Karma Runner executes the unit tests. Karma allows for units to be continually run in the background either against PhantomJS, simultaneously against a suite of browsers from the desktop, or remotely on virtual machines with SauceLabs. I've been making minor contributions to the project repository - mainly documentation and fixes.
- Integration. Protractor executes the integration test. Protractor leverages Selenium's WebDriver to load up the actual site, simulating interactions, and then test for expectations programatically. This works well from the desktop against basic browsers. In theory it also works with mobile browsers, but I couldn't get mobile to execute programtically so I had to rely on manual testing (I'm sure it's possible with greater investment of time). I've been making minor contributions to the project repository - mainly documentation and fixes.
Cross browser. SauceLabs has been integrated with Karma and Protractor to execute remotely against a suite of platforms and browsers.
- Karma has good native SauceLabs integration for queueing multiple tests at the same time, Protractor required a bit of custom development. For this, I used grunt-concurrent to run several tests in parallel with Sauce - eventually Protractor will hopefully natively support this parallelization like Karma does.
- After smoothing out a handful of compatibility issues in SauceLabs and Karma between various desktop drivers, the standard desktop browser tests finally ran smoothly. But the mobile web suite still had some kinks between various in-progress drivers that prohibited me from running the automated tests (this should clear one day).
- For interactive debugging sessions, SauceLabs also provides tunneling to remotely test the site running on your machine. In case I need it, it helps that I have VMware Fusion with a handful of Windows OS images. SauceLabs (or BrowserStack) simplify the interactive testing though because you don't need to worry about setting up your own virtual machine with Fusion - which can be time consuming and generally frustrating. If you can afford it, using SauceLabs/Browser stack for interactive testing is best.
Analysis. Grunt-Plato has also be integrated into my Grunt pipeline to produce automated JS code analysis.
Continuous Integration
Build.
- For continuous integration, Jenkins has been setup on my local machine to monitor my GIT repository, it runs the test suite, and sends me an email when the build passes/fails after a commit. This isn't entirely practical for a real project since it's running on my personal machine on a dedicated port, but I wanted to get my feet wet with Jenkins.
- Since moving the site's repository over to GitHub, I've now got TravisCI configured to run the Karma Javacript unit tests and send me pass/fail emails on commits. The project's build status on Travis is publically available.
Deploy. A grunt-s3 task programtically gzips and uploads my personalized builds to Amazon where the site is staticlly hosted. The demo site gets uploaded to GitHub Pages via grunt-gh-pages.